A quick introduction to XML and JSON
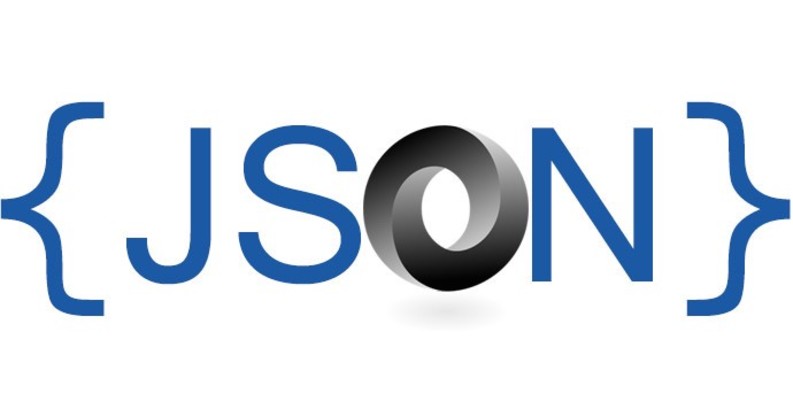
Moving forward with our web and mobile development tutorial series, here we'll be briefly introducing Extensible Markup Language (XML) and JavaScript Object Notation (JSON), both of which are commonly used with Application Programming Interfaces (APIs) to pass data back and forth between software programs (or to simply store data).
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
Imagine for a moment you had to invent a standardized notation by which to pass data — in text form — from one computer to another, where the data is most often in the form of nested objects (e.g. many blog posts that each have attributes such as author, date, etc., each with multiple comments that have their own author, date, etc.). How would you do it? How would you accommodate the hundreds of different variables and quirks so that your notation was stable? This is the problem that both XML and JSON solve.
EXTENSIBLE MARKUP LANGUAGE (XML)
Here is an example of what XML looks like, modified from this XML file on W3Schools:
<?xml version="1.0" encoding="ISO-8859-1"?>
<breakfast_menu>
<food>
<name>Belgian Waffles</name>
<price>$5.95</price>
<description>
two of our famous Belgian Waffles with
plenty of real maple syrup
</description>
<calories>650</calories>
</food>
<food>
<name>Strawberry Belgian Waffles</name>
<price>$7.95</price>
<description>
light Belgian waffles covered
with strawberries and whipped cream
</description>
<calories>900</calories>
<sides>
<side>
<name>Sausage</name>
<price>$2.00</price>
</side>
<side>
<name>Bacon</name>
<price>$2.50</price>
</side>
</sides>
</food>
</breakfast_menu>
Here we have a couple of menu items, where the second item has a couple of sides embedded along with it. The basic principle here is that, like HTML, XML starts out with a line declaring what version and what encoding the document has (there are tons of options), and it has tags that describe parts of the document, with content inside them.
XML can also be formatted to use tag attributes, which can tighten things up a bit. For example (borrowed from here):
<person firstName="John" lastName="Smith" age="25">
<address
streetAddress="21 2nd Street"
city="New York"
state="NY"
postalCode="10021" />
<phoneNumbers>
<phoneNumber type="home" number="212 555-1234"/>
<phoneNumber type="fax" number="646 555-4567"/>
</phoneNumbers>
</person>
That's pretty much all you need to know for now. XML isn't something to be scared of, it's just data formatted in a standardized way.
JAVASCRIPT OBJECT NOTATION
One of the biggest complaints about XML is that it's cumbersome — too many characters. This is generally true, but data can be compressed and formatted in such a way that XML and JSON are similar. Thus, JSON and XML really are interchangeable, though many modern developers prefer to use JSON.
The second example above in JSON could look like this:
{
"firstName": "John",
"lastName": "Smith",
"age": 25,
"address": {
"streetAddress": "21 2nd Street",
"city": "New York",
"state": "NY",
"postalCode": "10021"
},
"phoneNumber": [
{
"type": "home",
"number": "212 555-1234"
},
{
"type": "fax",
"number": "646 555-4567"
}
]
}
Notice that JSON is simply JavaScript objects, or hashes, with “keys” and “values.” This is the format we will be using in our tutorial series mostly from here forward, especially because Ruby on Rails makes it easy to setup JSON APIs for our web and mobile applications to use.
- — -
In our next post in this series we will be quickly introducing Backbone.js, a development framework with an MVC architecture kinda like Rails, which will conclude the “quick introductions” part of the series before we move on to discussing the specific application we will be building together. Previous post: CoffeeScript Introduction.