A quick introduction to Embedded Ruby (a.k.a. ERB, eRuby)
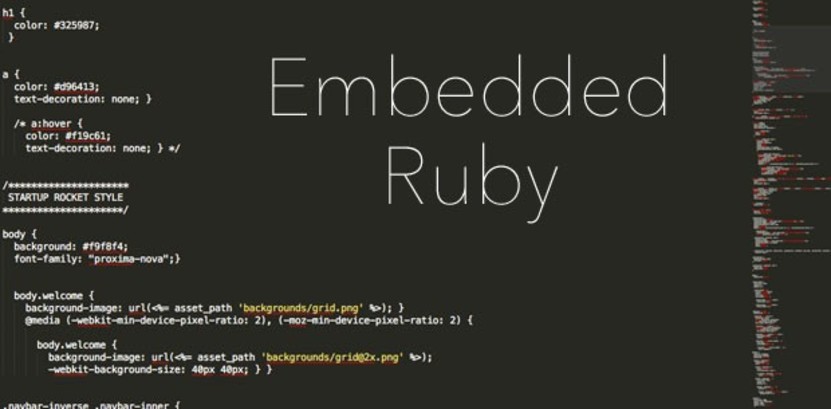
Before we dive directly into Ruby on Rails app development, after reading the Ruby introduction post in this series it is worth taking a moment to understand the concept and practice of Embedded Ruby.
[Author's note: I wrote the first couple dozen tutorials in this series a few years ago and I'm in the process of updating the content to reflect the evolution of best practices in the industry. Please comment if you see anything I missed that should be updated. Thanks!]
Assuming you have been following along with this tutorial series, or are already familiar with the basics of front- and back-end code, ERB is a convenient practice of embedding Ruby inside a front-end code document (e.g. a HTML file). For example:
<!DOCTYPE html>
<html>
<body>
<p>Hello, <%= user.first_name %>.</p>
</body>
</html>
A file such as this would end in .html.erb — but you could also have:
$("a").attr("href", "<%= user.blog_url %>")
Which would be a *.js.erb file (remember from our jQuery intro tutorial what this line would do?).
You get the idea… And a *.css.erb file would look something like this:
.navbar {
background-image: url(<%= asset_path 'logo.png' %>);
}
…where “asset_path” is a ruby helper method.
The overall idea with ERB is that the embedded Ruby is processed in the backend before being sent off to a requesting web browser. Thus, for the three examples above, what would actually be sent to the browser is something like:
<!DOCTYPE html>
<html>
<body>
<p>Hello, Jack.</p>
</body>
</html>
$("a").attr("href", "http://jacksblog.wordpress.com")
.navbar {
background-image: url(http://www.example.com/assets/logo.png);
}
WHY IS ERB USEFUL?
As you can hopefully extrapolate from the examples above, ERB allows web developers to embed information from a back-end database, or information otherwise calculated/retrieved from the server side of things. This is useful because instead of having a gazillion different files (“Hello Jack”, “Hello Ron”, etc…), you can just have one template file that says
“Hello <%= user.first_name %>”.
As we move next into introducing Ruby on Rails, know that this concept of embedding Ruby in a front-end document is extremely important — in fact, if you understand ERB and front-end code then you are well on your way to understanding conceptually how Rails makes things easier for web developers. For those who want to dive deeper into ERB, we'd recommend reading this online Ruby Doc page or this introduction to ERB templating.
- — -
Next post: Ruby on Rails introduction. Previous post: Ruby introduction.